Azure Bicep | Deploy Virtual Machines
For deploying Virtual Machines or resources in Azure there are different methods to use. Think about Terraform, PowerShell, ARM Templates or Azure Bicep. When you read the documentation of Microsoft:
Bicep is a domain-specific language (DSL) that uses declarative syntax to deploy Azure resources. In a Bicep file, you define the infrastructure you want to deploy to Azure, and then use that file throughout the development lifecycle to repeatedly deploy your infrastructure. Your resources are deployed in a consistent manner.
Bicep provides concise syntax, reliable type safety, and support for code reuse. Bicep offers a first-class authoring experience for your infrastructure-as-code solutions in Azure.
So deploying resources in Azure with Azure Bicep, you will get some benefits like described in the documentation. (link)
Goal
In this example we will start deploying some Virtual Machines. These Virtual Machines can be used for a testlab
Virtual Machine | Ip Address | Purpose |
DC01 | 10.10.0.4 | Domain Controller |
EX01 | 10.10.0.5 | Exchange Server |
WIN11 | 10.10.0.6 | Client for Access to servers over RDP |
So three virtual machines, network and network security groups, public ips and storage will be created with Azure Bicep.
Azure Bicep files
Azure Bicep is using it’s own file extension. Using VSCode can be usefull for auto completion on commands or creating the resources within the bicep file. We are going to create five files:
azuredeploy.parameters.json
In this JSON file we will define some variables that are generic and to use again withing the command of deployment.
{
"$schema": "https://schema.management.azure.com/schemas/2019-04-01/deploymentParameters.json#",
"contentVersion": "1.0.0.0",
"parameters": {
"vmAdminUserName": {
"value": "GetToTheCloudAdmin"
},
"vmAdminPassword": {
"value": "YOUROWNPASSWORD"
},
"addressPrefix": {
"value": "10.10.0.0/16"
},
"subnetName": {
"value": "subnet"
},
"subnetPrefix": {
"value": "10.10.0.0/24"
},
"virtualNetworkName": {
"value": "TestDomain-TestLab"
}
}
}
We define the JSON:
- vmAdminUserName
the local administrator username for the virtual machine - vmAdminPassword
the local administrator password for the user defined at vmAdminUserName - addressPrefix
the address prefix for the subnet - subnetName
name of the subnet - subnetPrefix
the address prefix within the subnet - virtualNetworkName
the name of the virtual network
dc.bicep
In every bicep file, you will define the resource that needs to be deployed. This will be the state of the initial deployment. Every time you deploy the bicep file, there will be a check if the contents of the bicep file van be found on Azure. Any changes to the bicep file will be a NEW action on Azure. Removing resources within the bicep file will not remove the resource from azure. Changing a name of a resource in the bicep file will not change the name of the resource on azure but will create a new resource with that name.
Visualizing the contents of a bicep file:
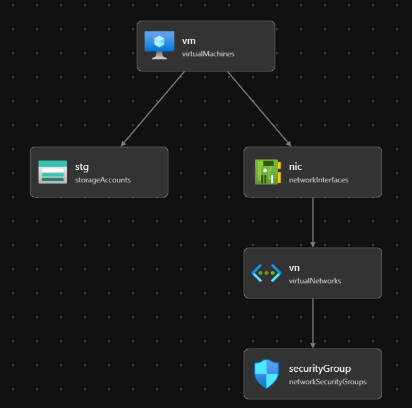
The contents:
@description('Username for the Virtual Machine.')
param vmAdminUserName string
@description('Password for the Virtual Machine.')
@minLength(12)
@secure()
param vmAdminPassword string
@description('The Windows version for the VM.')
@allowed([
'2022-datacenter'
'2022-datacenter-azure-edition-core'
'win11-22h2-avd'
])
param OSVersion string = '2022-datacenter'
@description('VM Publisher.')
@allowed([
'microsoftwindowsdesktop'
'MicrosoftWindowsServer'
])
param publisher string = 'MicrosoftWindowsServer'
@description('VM Offer.')
@allowed([
'WindowsServer'
'windows-11'
])
param vmOffer string = 'WindowsServer'
@description('Size of the virtual machine.')
param vmSize string = 'Standard_B2s'
@description('Location for all resources.')
param location string = resourceGroup().location
@description('Name of the virtual machine.')
param vmName string = 'DC01'
@description('Input from the parameters file')
param addressPrefix string
param subnetName string
param subnetPrefix string
param virtualNetworkName string
var storageAccountName = 'bootdiags${uniqueString(resourceGroup().id)}'
var nicName = 'NIC-DC01'
var networkSecurityGroupName = 'NSG-DC01'
// adding a storage account
resource stg 'Microsoft.Storage/storageAccounts@2021-04-01' = {
name: storageAccountName
location: location
sku: {
name: 'Standard_LRS'
}
kind: 'Storage'
}
// adding network security group to vnic with rules
resource securityGroup 'Microsoft.Network/networkSecurityGroups@2021-02-01' = {
name: networkSecurityGroupName
location: location
properties: {
securityRules: [
{
name: 'default-allow-5985'
properties: {
priority: 100
access: 'Allow'
direction: 'Inbound'
destinationPortRange: '5985'
protocol: 'Tcp'
sourcePortRange: '*'
sourceAddressPrefix: '*'
destinationAddressPrefix: '*'
}
}
]
}
}
// adding virtual network with parameters from parameter file
resource vn 'Microsoft.Network/virtualNetworks@2021-02-01' = {
name: virtualNetworkName
location: location
properties: {
addressSpace: {
addressPrefixes: [
addressPrefix
]
}
subnets: [
{
name: subnetName
properties: {
addressPrefix: subnetPrefix
networkSecurityGroup: {
id: securityGroup.id
}
}
}
]
}
}
// adding vnic to configuration
resource nic 'Microsoft.Network/networkInterfaces@2021-02-01' = {
name: nicName
location: location
properties: {
ipConfigurations: [
{
name: 'ipconfigDC01'
properties: {
subnet: {
id: resourceId('Microsoft.Network/virtualNetworks/subnets', vn.name, subnetName)
}
}
}
]
}
}
// adding virtual machine
resource vm 'Microsoft.Compute/virtualMachines@2021-03-01' = {
name: vmName
location: location
properties: {
hardwareProfile: {
vmSize: vmSize
}
osProfile: {
computerName: vmName
adminUsername: vmAdminUserName
adminPassword: vmAdminPassword
}
storageProfile: {
imageReference: {
publisher: publisher
offer: vmOffer
sku: OSVersion
version: 'latest'
}
osDisk: {
createOption: 'FromImage'
caching: 'ReadWrite'
managedDisk: {
storageAccountType: 'StandardSSD_LRS'
}
}
dataDisks: [
{
diskSizeGB: 1023
lun: 0
createOption: 'Empty'
}
]
}
networkProfile: {
networkInterfaces: [
{
id: nic.id
}
]
}
diagnosticsProfile: {
bootDiagnostics: {
enabled: true
storageUri: stg.properties.primaryEndpoints.blob
}
}
}
}
ex.bicep
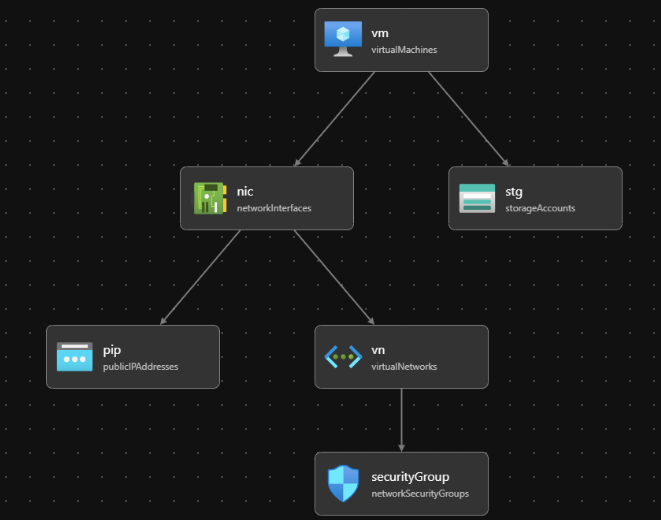
@description('Username for the Virtual Machine.')
param vmAdminUserName string
@description('Password for the Virtual Machine.')
@minLength(12)
@secure()
param vmAdminPassword string
@description('Unique DNS Name for the Public IP used to access the Virtual Machine.')
param dnsLabelPrefix string = toLower('${vmName}-${uniqueString(resourceGroup().id, vmName)}')
@description('Name for the Public IP used to access the Virtual Machine.')
param publicIpName string = 'PublicIP-EX01'
@description('Allocation method for the Public IP used to access the Virtual Machine.')
@allowed([
'Dynamic'
'Static'
])
param publicIPAllocationMethod string = 'Dynamic'
@description('SKU for the Public IP used to access the VM.')
@allowed([
'Basic'
'Standard'
])
param publicIpSku string = 'Basic'
@description('The Windows version for the VM.')
@allowed([
'2022-datacenter'
'2022-datacenter-azure-edition-core'
'win11-22h2-avd'
])
param OSVersion string = '2022-datacenter'
@description('VM Publisher.')
@allowed([
'microsoftwindowsdesktop'
'MicrosoftWindowsServer'
])
param publisher string = 'MicrosoftWindowsServer'
@description('VM Offer.')
@allowed([
'WindowsServer'
'windows-11'
])
param vmOffer string = 'WindowsServer'
@description('Size of the virtual machine.')
param vmSize string = 'Standard_B2s'
@description('Location for all resources.')
param location string = resourceGroup().location
@description('Name of the virtual machine.')
param vmName string = 'EX01'
@description('Input from the parameters file')
param addressPrefix string
param subnetName string
param subnetPrefix string
param virtualNetworkName string
var storageAccountName = 'bootdiags${uniqueString(resourceGroup().id)}'
var nicName = 'NIC-EX01'
var networkSecurityGroupName = 'NSG-EX01'
// adding a storage account
resource stg 'Microsoft.Storage/storageAccounts@2021-04-01' = {
name: storageAccountName
location: location
sku: {
name: 'Standard_LRS'
}
kind: 'Storage'
}
// adding a public ip address
resource pip 'Microsoft.Network/publicIPAddresses@2021-02-01' = {
name: publicIpName
location: location
sku: {
name: publicIpSku
}
properties: {
publicIPAllocationMethod: publicIPAllocationMethod
dnsSettings: {
domainNameLabel: dnsLabelPrefix
}
}
}
// adding network security group to vnic with rules: 5985 for remote powershell and 443 for https inbound
resource securityGroup 'Microsoft.Network/networkSecurityGroups@2021-02-01' = {
name: networkSecurityGroupName
location: location
properties: {
securityRules: [
{
name: 'default-allow-5985'
properties: {
priority: 100
access: 'Allow'
direction: 'Inbound'
destinationPortRange: '5985'
protocol: 'Tcp'
sourcePortRange: '*'
sourceAddressPrefix: '*'
destinationAddressPrefix: '*'
}
}
{
name: 'default-allow-443'
properties: {
priority: 101
access: 'Allow'
direction: 'Inbound'
destinationPortRange: '443'
protocol: 'Tcp'
sourcePortRange: '*'
sourceAddressPrefix: '*'
destinationAddressPrefix: '*'
}
}
]
}
}
// adding virtual network with parameters from parameter file
resource vn 'Microsoft.Network/virtualNetworks@2021-02-01' = {
name: virtualNetworkName
location: location
properties: {
addressSpace: {
addressPrefixes: [
addressPrefix
]
}
subnets: [
{
name: subnetName
properties: {
addressPrefix: subnetPrefix
networkSecurityGroup: {
id: securityGroup.id
}
}
}
]
}
}
// adding vnic to configuration
resource nic 'Microsoft.Network/networkInterfaces@2021-02-01' = {
name: nicName
location: location
properties: {
ipConfigurations: [
{
name: 'ipconfigEX01'
properties: {
privateIPAllocationMethod: 'Dynamic'
publicIPAddress: {
id: pip.id
}
subnet: {
id: resourceId('Microsoft.Network/virtualNetworks/subnets', vn.name, subnetName)
}
}
}
]
}
}
// adding virtual machine
resource vm 'Microsoft.Compute/virtualMachines@2021-03-01' = {
name: vmName
location: location
properties: {
hardwareProfile: {
vmSize: vmSize
}
osProfile: {
computerName: vmName
adminUsername: vmAdminUserName
adminPassword: vmAdminPassword
}
storageProfile: {
imageReference: {
publisher: publisher
offer: vmOffer
sku: OSVersion
version: 'latest'
}
osDisk: {
createOption: 'FromImage'
caching: 'ReadWrite'
managedDisk: {
storageAccountType: 'StandardSSD_LRS'
}
}
dataDisks: [
{
diskSizeGB: 1023
lun: 0
createOption: 'Empty'
}
]
}
networkProfile: {
networkInterfaces: [
{
id: nic.id
}
]
}
diagnosticsProfile: {
bootDiagnostics: {
enabled: true
storageUri: stg.properties.primaryEndpoints.blob
}
}
}
}
// outputting deployment result and dns url for connection
output hostname string = pip.properties.dnsSettings.fqdn
win11.bicep
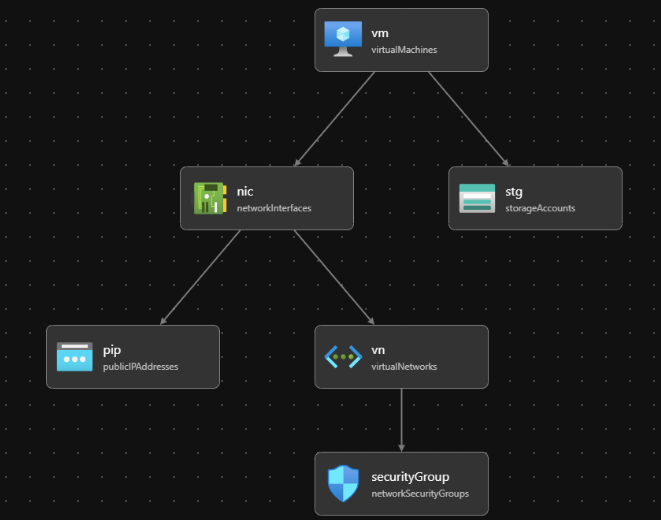
@description('Username for the Virtual Machine.')
param vmAdminUserName string
@description('Password for the Virtual Machine.')
@minLength(12)
@secure()
param vmAdminPassword string
@description('Unique DNS Name for the Public IP used to access the Virtual Machine.')
param dnsLabelPrefix string = toLower('${vmName}-${uniqueString(resourceGroup().id, vmName)}')
@description('Name for the Public IP used to access the Virtual Machine.')
param publicIpName string = 'PublicIP-WIN11'
@description('Allocation method for the Public IP used to access the Virtual Machine.')
@allowed([
'Dynamic'
'Static'
])
param publicIPAllocationMethod string = 'Dynamic'
@description('SKU for the Public IP used to access the VM.')
@allowed([
'Basic'
'Standard'
])
param publicIpSku string = 'Basic'
@description('The Windows version for the VM.')
@allowed([
'2022-datacenter'
'2022-datacenter-azure-edition-core'
'win11-22h2-avd'
])
param OSVersion string = 'win11-22h2-avd'
@description('VM Publisher.')
@allowed([
'microsoftwindowsdesktop'
'MicrosoftWindowsServer'
])
param publisher string = 'microsoftwindowsdesktop'
@description('VM Offer.')
@allowed([
'WindowsServer'
'windows-11'
])
param vmOffer string = 'windows-11'
@description('Size of the virtual machine.')
param vmSize string = 'Standard_B2s'
@description('Location for all resources.')
param location string = resourceGroup().location
@description('Name of the virtual machine.')
param vmName string = 'WIN11'
@description('Input from the parameters file')
param addressPrefix string
param subnetName string
param subnetPrefix string
param virtualNetworkName string
var storageAccountName = 'bootdiags${uniqueString(resourceGroup().id)}'
var nicName = 'NIC-WIN11'
var networkSecurityGroupName = 'NSG-WIN11'
// adding a storage account
resource stg 'Microsoft.Storage/storageAccounts@2021-04-01' = {
name: storageAccountName
location: location
sku: {
name: 'Standard_LRS'
}
kind: 'Storage'
}
// adding a public ip address
resource pip 'Microsoft.Network/publicIPAddresses@2021-02-01' = {
name: publicIpName
location: location
sku: {
name: publicIpSku
}
properties: {
publicIPAllocationMethod: publicIPAllocationMethod
dnsSettings: {
domainNameLabel: dnsLabelPrefix
}
}
}
// adding network security group to vnic with rules: 5985 for remote powershell and 443 for https inbound
resource securityGroup 'Microsoft.Network/networkSecurityGroups@2021-02-01' = {
name: networkSecurityGroupName
location: location
properties: {
securityRules: [
{
name: 'default-allow-3389'
properties: {
priority: 100
access: 'Allow'
direction: 'Inbound'
destinationPortRange: '3389'
protocol: 'Tcp'
sourcePortRange: '*'
sourceAddressPrefix: '*'
destinationAddressPrefix: '*'
}
}
]
}
}
// adding virtual network with parameters from parameter file
resource vn 'Microsoft.Network/virtualNetworks@2021-02-01' = {
name: virtualNetworkName
location: location
properties: {
addressSpace: {
addressPrefixes: [
addressPrefix
]
}
subnets: [
{
name: subnetName
properties: {
addressPrefix: subnetPrefix
networkSecurityGroup: {
id: securityGroup.id
}
}
}
]
}
}
// adding vnic to configuration
resource nic 'Microsoft.Network/networkInterfaces@2021-02-01' = {
name: nicName
location: location
properties: {
ipConfigurations: [
{
name: 'ipconfigWIN11'
properties: {
privateIPAllocationMethod: 'Dynamic'
publicIPAddress: {
id: pip.id
}
subnet: {
id: resourceId('Microsoft.Network/virtualNetworks/subnets', vn.name, subnetName)
}
}
}
]
}
}
// adding virtual machine
resource vm 'Microsoft.Compute/virtualMachines@2021-03-01' = {
name: vmName
location: location
properties: {
hardwareProfile: {
vmSize: vmSize
}
osProfile: {
computerName: vmName
adminUsername: vmAdminUserName
adminPassword: vmAdminPassword
}
storageProfile: {
imageReference: {
publisher: publisher
offer: vmOffer
sku: OSVersion
version: 'latest'
}
osDisk: {
createOption: 'FromImage'
caching: 'ReadWrite'
managedDisk: {
storageAccountType: 'StandardSSD_LRS'
}
}
dataDisks: [
{
diskSizeGB: 1023
lun: 0
createOption: 'Empty'
}
]
}
networkProfile: {
networkInterfaces: [
{
id: nic.id
}
]
}
diagnosticsProfile: {
bootDiagnostics: {
enabled: true
storageUri: stg.properties.primaryEndpoints.blob
}
}
}
}
// outputting deployment result and dns url for connection
output hostname string = pip.properties.dnsSettings.fqdn
bicep-deploy.ps1
For deploying the bicep files, you need to use a PowerShell script. This script is providing the access to Azure after logging in and start the deployment.
# connecting to azure
Connect-AZAccount
# setting variables for resource gorup
$LocationName = "westeurope"
$ResourceGroupName = "TestDomain"
## creating resource group
Try {
$newGroup = New-AzResourceGroup -Name $ResourceGroupName -Location $LocationName -InformationAction SilentlyContinue
Write-host "[SUCCESS] Resource Group is created with the name $($ResourceGroupName)" -ForegroundColor Green
}
catch {
Write-Host "[ERROR] Creating Resource group" -ForegroundColor Red
}
After creating the resource group, this group can be used for the deployment of the resources.
# creating deployment name
$date = Get-Date -Format "MM-dd-yyyy"
$rand = Get-Random -Maximum 1000
$deploymentName = "DeploymentDC-"+"$date"+"-"+"$rand"
# starting deployment
New-AzResourceGroupDeployment -Name $deploymentName -ResourceGroupName $ResourceGroupName -TemplateFile ".\DC.bicep" -TemplateParameterFile ".\azuredeploy.parameters.json"
New-AzResourceGroupDeployment -Name $deploymentName -ResourceGroupName $ResourceGroupName -TemplateFile ".\EX.bicep" -TemplateParameterFile ".\azuredeploy.parameters.json"
New-AzResourceGroupDeployment -Name $deploymentName -ResourceGroupName $ResourceGroupName -TemplateFile ".\WIN11.bicep" -TemplateParameterFile ".\azuredeploy.parameters.json"
After deployment
Browsing to the portal of Azure and looking for the resource group we will see now all the resources as described in the bicep files.
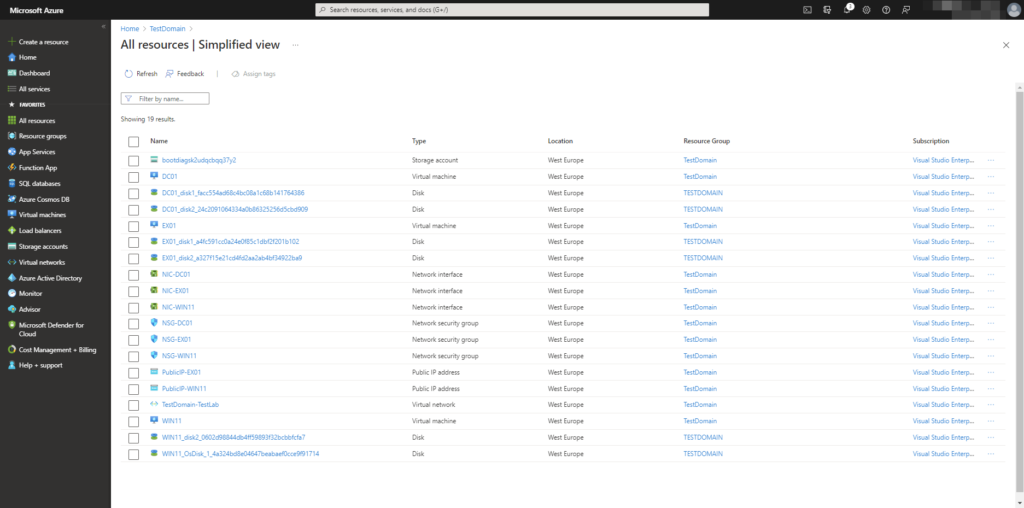
Three virtual machines in a virtual network. EX01 and WIN11 provided with also an external IP address and for each virtual machine a vNic with an internal address.